Set the controls data context to the object that has the data, then bind specific properties.
Lets assume that you have a class User that has a property IsActive and you want to bind a checkbox to user.IsActive:
In code set the data context (note you can limit the scope to a particular control).
this.DataContext = user;
bind in XAML:
<CheckBox IsChecked="{Binding Path=IsActive}"/>
Here's a sample that has a simple user control with a couple of textboxes and checkboxes that use databinding to display data. The control pretends to need some user info. It stores it in one of its properties "CurrentUser". Once the property is populated it, the controls will display it:
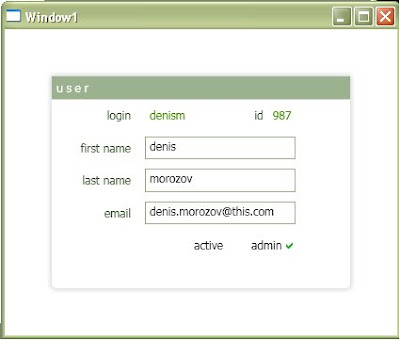
First lets create a data container in the form of a class "User".
namespace BindingSample
{
public class User
{
public int Id { get; set; }
public bool IsActive { get; set; }
public bool IsAdmin { get; set; }
public string UserName { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public string Email { get; set; }
}
}
Then lets create a XAML UserControl - the main thing to note here is the binding code (in yellow):
In control's code, lets create a property CurrentUser, there we can set the data context:
<UserControl x:Class="BindingSample.formUser"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml">
<Border CornerRadius="5" Margin="50" Background="White">
<Border.BitmapEffect>
<OuterGlowBitmapEffect GlowColor="LightGray"/>
</Border.BitmapEffect>
<StackPanel>
<!-- header -->
<Grid Background="#8AA37B" Opacity=".85">
<StackPanel Orientation="Horizontal">
<Label Foreground="White" FontSize="12">u s e r</Label>
</StackPanel>
</Grid>
<!-- body -->
<Grid >
<Grid.ColumnDefinitions>
<ColumnDefinition Width="10"/>
<ColumnDefinition Width="69*" />
<ColumnDefinition Width="70*" />
<ColumnDefinition Width="35*" />
<ColumnDefinition Width="35*" />
<ColumnDefinition Width="35*" />
<ColumnDefinition Width="10" />
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
</Grid.RowDefinitions>
<Label Grid.Row="0" Grid.Column="1" HorizontalContentAlignment="Right" Margin="5" Foreground="#435D36" FontSize="12">login</Label>
<StackPanel Grid.Row="0" Grid.Column="3" Grid.ColumnSpan="2" Margin="5" Orientation="Horizontal" FlowDirection="RightToLeft">
<Label Content="{Binding Path=Id}" Foreground="#458B00" FontSize="12"></Label>
<Label Foreground="#435D36" FontSize="12">id</Label>
</StackPanel>
<Label Grid.Row="1" Grid.Column="1" HorizontalContentAlignment="Right" Margin="5" Foreground="#435D36" FontSize="12">first name</Label>
<Label Grid.Row="2" Grid.Column="1" HorizontalContentAlignment="Right" Margin="5" Foreground="#435D36" FontSize="12">last name</Label>
<Label Grid.Row="3" Grid.Column="1" HorizontalContentAlignment="Right" Margin="5" Foreground="#435D36" FontSize="12">email</Label>
<Label Grid.Row="0" Grid.Column="2" Margin="5" Content="{Binding Path=UserName}" Foreground="#458B00" FontSize="12"></Label>
<TextBox Grid.Row="1" Grid.Column="2" Margin="5" Text="{Binding Path=FirstName}" Grid.ColumnSpan="3" BorderBrush="#8FA880" FontSize="12"></TextBox>
<TextBox Grid.Row="2" Grid.Column="2" Margin="5" Text="{Binding Path=LastName}" Grid.ColumnSpan="3" BorderBrush="#8FA880" FontSize="12"></TextBox>
<TextBox Grid.Row="3" Grid.Column="2" Margin="5" Text="{Binding Path=Email}" Grid.ColumnSpan="3" BorderBrush="#8FA880" FontSize="12"></TextBox>
<StackPanel Orientation="Horizontal" Grid.Row="6" Grid.Column="1" Grid.ColumnSpan="4" Margin="5" FlowDirection="RightToLeft">
<CheckBox Margin="0,5,15,5" BorderBrush="Transparent" Background="Transparent" FlowDirection="RightToLeft" IsChecked="{Binding Path=IsAdmin}" FontSize="12" >admin</CheckBox>
<CheckBox Margin="0,5,5,5" BorderBrush="Transparent" Background="Transparent" FlowDirection="RightToLeft" IsChecked="{Binding Path=IsActive}" FontSize="12" >active</CheckBox>
</StackPanel>
</Grid>
</StackPanel>
</Border>
</UserControl>
Next step is to create a window and instanciate the control that was created above: (create a new XAML file) and add the control:
namespace BindingSample
{
/// <summary>
/// Interaction logic for formUser.xaml
/// </summary>
public partial class formUser : UserControl
{
private User currentUser = new User();
public formUser()
{
InitializeComponent();
}
public User CurrentUser
{
get { return this.currentUser; }
set
{
this.currentUser = value;
this.DataContext = currentUser;
}
}
}
}
<Window x:Class="BindingSample.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:BindingSample"
Title="Window1" Height="300" Width="300">
<Grid>
<local:formUser x:Name="formUserDetail"/>
</Grid>
</Window>
And in code behind, lets populate the user and pass it to the control:
namespace BindingSample
{
/// <summary>
/// Interaction logic for Window1.xaml
/// </summary>
public partial class Window1 : Window
{
private User user;
public Window1()
{
/*create a user*/
user = new User();
user.Id = 987;
user.IsActive = false;
user.IsAdmin = true;
user.UserName = "denism";
user.FirstName = "denis";
user.LastName = "morozov";
user.Email = "denis.morozov@this.com";
InitializeComponent();
this.formUserDetail.CurrentUser = user;
}
}
}
No comments:
Post a Comment